打开ExpenseEntryItemList.js并从 redux 库导入连接。
import { connect } from 'react-redux';
接下来,导入 addExpenseList 和 deleteExpense 操作。
import { getExpenseList, deleteExpense } from '../actions/expenseActions';
接下来,添加带有道具的构造函数。
constructor(props) { super(props); }
接下来,在componentDidMount()生命周期中调用getExpenseList 。
componentDidMount() { this.props.getExpenseList(); }
接下来,编写一个方法来处理删除费用选项。
handleDelete = (id,e) => { e.preventDefault(); this.props.deleteExpense(id); }
现在,让我们编写一个函数 getTotal 来计算总费用。
getTotal() { let total = 0; if (this.props.expenses != null) { for (var i = 0; i < this.props.expenses.length; i++) { total += this.props.expenses[i].amount } } return total; }
接下来,更新渲染方法并列出费用项目。
render() { let lists = []; if (this.props.expenses != null) { lists = this.props.expenses.map((item) => <tr key={item.id}> <td>{item.name}</td> <td>{item.amount}</td> <td>{new Date(item.spendDate).toDateString()}</td> <td>{item.category}</td> <td><a href="#" onClick={(e) => this.handleDelete(item.id, e)}>Remove</a></td> </tr> ); } return ( <div> <table> <thead> <tr> <th>Item</th> <th>Amount</th> <th>Date</th> <th>Category</th> <th>Remove</th> </tr> </thead> <tbody> {lists} <tr> <td colSpan="1" style={{ textAlign: "right" }}>Total Amount</td> <td colSpan="4" style={{ textAlign: "left" }}> {this.getTotal()} </td> </tr> </tbody> </table> </div> ); }
接下来,编写mapStateToProps和mapDispatchToProps方法。
const mapStateToProps = state => { return { expenses: state.data }; }; const mapDispatchToProps = { getExpenseList, deleteExpense };
在这里,我们将 redux store 中的费用项映射到费用属性,并将 distpatcher、getExpenseList和deleteExpense 附加到组件属性。
最后,使用 connect api 将组件连接到 Redux 存储。
export default connect( mapStateToProps, mapDispatchToProps )(ExpenseEntryItemList);
该应用程序的完整源代码如下 –
import React from "react"; import { connect } from 'react-redux'; import { getExpenseList, deleteExpense } from '../actions/expenseActions'; class ExpenseEntryItemList extends React.Component { constructor(props) { super(props); } componentDidMount() { this.props.getExpenseList(); } handleDelete = (id, e) => { e.preventDefault(); this.props.deleteExpense(id); } getTotal() { let total = 0; if (this.props.expenses != null) { for (var i = 0; i < this.props.expenses.length; i++) { total += this.props.expenses[i].amount } } return total; } render() { let lists = []; if (this.props.expenses != null) { lists = this.props.expenses.map((item) => <tr key={item.id}> <td>{item.name}</td> <td>{item.amount}</td> <td>{new Date(item.spendDate).toDateString()}</td> <td>{item.category}</td> <td><a href="#" onClick={(e) => this.handleDelete(item.id, e)}>Remove</a> </td> </tr> ); } return ( <div> <table> <thead> <tr> <th>Item</th> <th>Amount</th> <th>Date</th> <th>Category</th> <th>Remove</th> </tr> </thead> <tbody> {lists} <tr> <td colSpan="1" style={{ textAlign: "right" }}>Total Amount</td> <td colSpan="4" style={{ textAlign: "left" }}> {this.getTotal()} </td> </tr> </tbody> </table> </div> ); } } const mapStateToProps = state => { return { expenses: state.data }; }; const mapDispatchToProps = { getExpenseList, deleteExpense }; export default connect( mapStateToProps, mapDispatchToProps )(ExpenseEntryItemList);
接下来,使用 npm 命令为应用程序提供服务。
npm start
接下来,打开浏览器,在地址栏输入http://localhost:3000,回车。
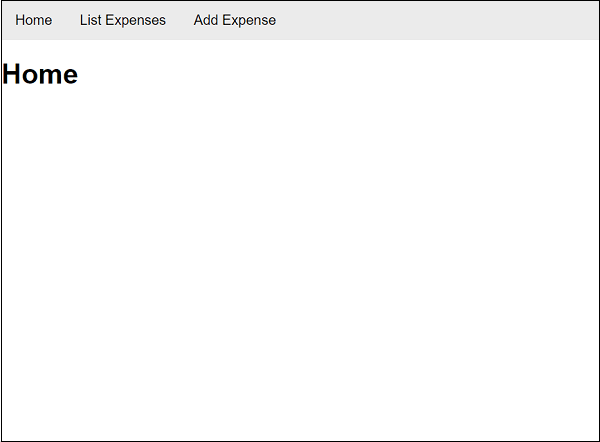