使用一维数组非常棒,但有时您需要的不止于此。为了在 Javascript 中存储更复杂的数据结构,我们使用对象。对象允许我们同时拥有数据的键和对。
如何制作一个对象#
在 Javascript 中创建对象就像创建数组一样简单。我们使用大括号来定义我们的对象,然后有键/值对。一个简单的对象如下所示:
let myObject = { "someKey" : "someValue", "someOtherKey" : "someOtherValue" };
对象通过允许我们给它们 keys 来为我们提供关于我们的价值观的背景。对象可以是多维的,这仅意味着它们自身包含对象。它们还可以包含数组。因此,下面也是一个有效的对象:
let myObject = { "someKey" : { "subKey" : "aValue", "anotherKey" : "anotherValue" }, "someOtherKey": [ 1, 2, 3, 4, 5, 6 ], "andFinally" : "oneMoreValue" }
对象内同一级别的对象键必须是唯一的,因此您不能有重复的键。
其他定义对象的方法
上面的方法是我们最容易定义一个对象的方法,但你也可以这样做:
let myObject = new Object(); myObject.someKey = "someValue";
上面,我们最终得到一个具有一个键的对象,称为“ someKey ”,值为“ someValue ”。
访问对象的一部分#
由于对象有键,我们可以使用点 ( . ) 或方括号 ( [] ) 直接访问它们。让我们以我们的第一个对象为例:
let myObject = { "someKey" : { "subKey" : "aValue", "anotherKey" : "anotherValue" }, "someOtherKey": [ 1, 2, 3, 4, 5, 6 ], "andFinally" : "oneMoreValue" } // Both of these are the same way to access "someKey" in "myObject" // As such, they both return "aValue" let getMyKey = myObject.someKey; let anotherWay = myObject["someKey"]; // If we want to get an object multiple layers deep, we can use multiple // square brackets or dots: let subKey = myObject.someKey.subKey; let sameSubKey = myObject["someKey"]["subKey"];
例如,您可能会注意到这种格式模仿了我们在其他地方使用的格式document.querySelectorAll
。那是因为文档是一个包含函数的对象!实际上,您可以通过简单地将其记录在控制台中来找到对象window
上的所有功能:document
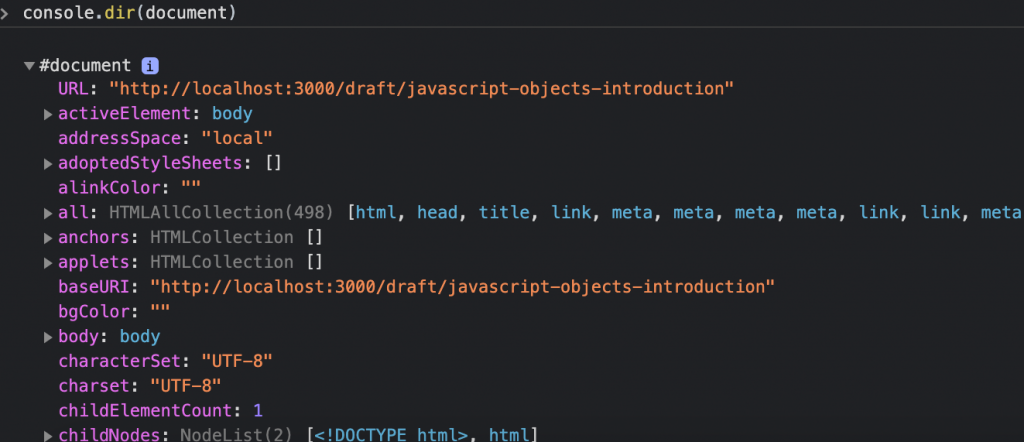
我们可以向我们的对象添加函数并调用它们:
let myObject = { runFunction: function(name) { console.log(`My name is ${name}`); } }; // Console logs "My name is John" myObject.runFunction("John");
Javascript 通常被描述为一种基于原型的语言。这里的原型指的是对象,我们这样称呼它是因为 Javascript 历史上没有使用过类,因此依赖于这样的对象。
遍历对象#
由于我们在 Javascript 对象中同时拥有键和值,因此访问对象中的每个项目变得有点棘手。最简单的方法之一是使用 for 循环:
let myObject = { "someKey" : "someValue", "someOtherKey" : "someOtherValue" }; for(const prop in myObject) { let key = prop; // "prop" here refers to the key name. let value = myObject[prop] // We can now use "prop" to refer to get the value }
获取所有键或值的另一种方法是使用Object.keys
and Object.values
。这两个都接受一个对象,并将其所有键或所有值隐藏到一个数组中。
一旦我们将它们放在一个数组中,我们就可以在我们的数组上使用 forEach。例如:
let myObject = { "someKey" : "someValue", "someOtherKey" : "someOtherValue" }; Object.keys(myObject).forEach(function(item) { console.log(item); // This will console log both "someKey" and "someOtherKey" let value = myObject[item]; // This will give us the value for both "someKey" and "someOtherKey" }); let valueArray = Object.values(myObject); // This returns [ "someValue", "someOtherValue" ]
使用对象创建功能原型#
我们已经谈到了 Javascript 通常如何被描述为一种原型语言。这意味着它基于对象的结构。在其他文章中,我们谈到了函数的工作原理。
原型本质上是 Javascript 如何实现在其他语言中称为类的功能。我们使用一个基本函数并扩展它的原型来为我们提供与该函数相关的函数。在下面的例子中:
- 我们有一个名为Engine的函数。
- Engine是一个对象,因此它有一些相关的功能。对于我们的示例,我们有一个名为go的相关函数。
- 一个Engine可以go,所以我们将go函数添加到我们的Engine原型中。
- 然后我们可以运行我们的 go 函数,使用在我们的Engine函数中定义的变量,我们已经用this关键字定义了这些变量。
- 由于变量与函数go位于同一对象上,因此可以从go函数中访问它们。
这是我们示例的代码:
let Engine = function(carName) { this.horsePower = 100000; this.speedMph = 10000000; this.carName = carName; } Engine.prototype.go = function() { console.log(`${this.carName} is going at ${this.speedMph}mph!`); } // This means we'll be able to more easily call our functions with Engine.go, rather than Engine.prototype.go Engine.prototype.constructor = Engine; let myCarEngine = new Engine('Marty McFly'); // This will console log 'Marty McFly is going at 10000000mph!' myCarEngine.go();
高级对象函数#
与数组一样,有许多复杂的方法来操作、访问和更改对象。因此,我们制作了另一篇文章来介绍这一点。
单击下方以了解有关更高级对象函数的更多信息。
学习:Everything Objects 单击此处查看操作对象的高级方法。