抽象的
在 C/C++ 程序中反转一个数字意味着交换数字,即将给定数字的最后一个数字带到第一个位置,反之亦然。
文章范围
- 本文提供了有关反转数字及其算法的基本理解。
- 在 C 中实现相同的不同方法。
先决条件
- 应该对 C/C++ 有基本的了解
- 了解循环和递归的工作原理。
介绍
正如我们所知,反转数字意味着交换数字,以便数字的最后一位在前,反之亦然。
让我们借助一个示例来理解相同的内容:-
给定输入是 56897。
然后给定数字的倒数将是 79865。
既然我们已经理解了反转数字的含义,让我们进一步了解它的算法。
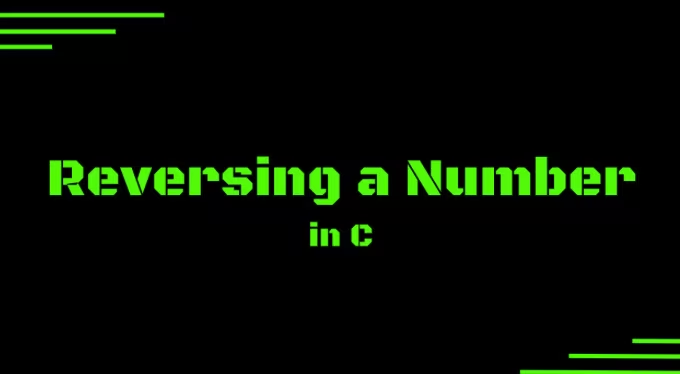
在 C 中反转数字的算法
第 1 步。让我们考虑给定的数字是 num = 5689。考虑反向数为rev_num =0。
步骤 2。现在我们将使用给定的公式,即。
rev_num = rev_num*10 + num%10 ;
数=数/ 10 ;
正如我们所看到的,每次我们提取给定数字的最后一位数字并通过将其乘以10来容纳rev_num中的最后一位数字。
为了避免从 num 中提取相同的最后一位数字,我们将通过将 num 除以10并将该值存储在num 中来更新 num。
步骤 3。我们将继续这个过程,直到给定的数字变得小于或等于0。
一旦我们到达那个阶段,我们就获得了我们的反向号码。
在上面的例子中,在第 5 次迭代之后,我们将获得我们的反向数字,即79865。
从 num 中提取的数字 | 乘以 10 即 rev_num |
---|---|
7 | 0*10+ 7 =7 |
9 | 7*10 +9 = 79 |
8 | 79*10+8 = 798 |
6 | 798*10+6=7986 |
5 | 7986*10+5=79865 |
在 C 中实现相同的不同方法
这里我们使用 2 个算术运算符,即模和除法运算符。
反转数字的迭代方法
# include <stdio.h>
int main(){
int num, rev_num = 0 , rem ;
prinf("enter the number you wish to reverse:");
scanf ("%d",&num);
// using while loop and decreasing num till num >= 0
while (num != 0){
rem = num %10 ;
rev_num = (rev_num *10) + rem;
num = num/ 10 ; //updating the the number by dividing it by 10
}
printf ("reverse of the given number %d is : %d", num , rev_num);
return 0;
}
输出
enter the number you wish to reverse: 54689
reverse of the given number 54689 is : 98645
反转数字的递归实现
# include <stdio.h>
int reversenumber(int n, int revnum){
if (n==0) return revnum;
return reversenumber (n/10 , n % 10 + k*10);
}
int main(){
int num, rev_num ;
prinf("enter the number you wish to reverse");
scanf ("%d",&num);
//calling recursive function reversenumber
rev_num = reversenumber(num, 0);
printf ("reverse of the given number %d is : %d", num , rev_num);
return 0;
}
输出
enter the number you wish to reverse: 54689
reverse of the given number 54689 is : 98645
解释
- 在递归函数中,
revnum
表示给定数字的倒数。 - 我们设置了一个条件,如果“n”表示的给定数字变为零,那么我们将返回我们的反向数字。
- 在所有其他情况下,我们将调用
reversenumber
(n/10, n % 10 + k*10) 并revnum
分别更新 n 和
迭代 | n 的值 | revnum 的值 | 返回值 |
---|---|---|---|
0 | 54689 | 0 | 返回(54689,0) |
1 | 5468 | 9 | 返回(5468,9) |
2 | 546 | 98 | 返回(546,98) |
3 | 54 | 986 | 返回 (54,986) |
4 | 5 | 9864 | 返回(5,9864) |
5 | 0 | 98654 | 当 n==0 时,我们将简单地返回 revnum = 98654 的值 |
使用功能
# include <stdio.h>
int revnumber( int n){
int rem , rev_num =0;
for (; n> 0; n= n/10){
rem = n%10 ;
rev_num = rev_num*10 + rem;
}
return rev_num;
}
int main(){
int num, rev_num = 0 ;
prinf("enter the number you wish to reverse:");
scanf ("%d",&num);
rev_num = revnumber (num);
printf ("reverse of the given number %d is : %d", num , rev_num);
return 0;
}
输出
enter the number you wish to reverse: 56897
reverse of the given number 54689 is : 79865
如果输入一个负数怎么办?
正如我们所知,计算机只处理二进制数,不像人类可以解释多种语言。负数的二进制数表示不同于正数。
因此,如果我们直接将上述算法应用于负数,则会产生错误的答案。为了避免这种情况,我们将所述负数乘以 -1 使其成为正数,然后应用算法将其反转,然后再次将 -1 与反转数相乘。
给定的代码将有助于更好地理解这一点。
# include <stdio.h>
# include <stdbool.h>
int main(){
int num, rev_num = 0 , rem ;
bool neg = false;
prinf("enter the number you wish to reverse:");
scanf ("%d",&num);
if (num < 0){
num = -num ;
neg = true;
}
// using while loop and decreasing num till num >= 0
while (num != 0){
rem = num %10 ;
rev_num = (rev_num *10) + rem;
num = num/ 10 ; //updating the the number by dividing it by 10
}
if (neg){
num = - num;
}
printf ("reverse of the given number %d is : %d", num , rev_num);
return 0;
}
在反转数字时处理溢出情况
正如我们所知,整数最多可以处理 32 位。可能会发生数字的反转可能超过或溢出 32 位。在这种情况下,每次检查反转数是否超过 32 位。
下面的代码提供了更好的理解。
# include <stdio.h>
int revnumber( int n){
int rem , rev_num =0, prev_num = 0;
while (n ! =0 ){
rem = n%10 ;
prev_num = (rev_num) *10 + rem;
if ((prev_num - rem )/10 != rev){
printf("WARNING REVERSE NUMBER IS OVERFLOWED!!!");
return 0;
}
else rev_num = prev_num;
n= n/10;
}
return rev_num;
}
int main(){
int num, rev_num = 0 ;
prinf("enter the number you wish to reverse:");
scanf ("%d",&num);
rev_num = revnumber (num);
if (rev_num != 0){
printf ("reverse of the given number %d is : %d", num , rev_num);
}
return 0;
}
在这段代码中,我们检查了倒数是否溢出。在溢出的情况下,rev_num将包含垃圾值,它不会等于 ( prev_num – rem )/ 10。
算法分析
时间复杂度
While 循环或递归函数针对 number 中存在的位数执行程序。数 num 中的多个数字以 10 为底计算 log (num)。因此时间复杂度为 O(log10(num))。
空间复杂度
我们没有使用任何额外的空间。因此空间复杂度为 O(1)。
结论
在本文中,我们已经看到了反转数字的算法和不同的方法,通过这些方法可以在类似 C 的循环中递归地实现它。
除此之外,我们还可以看到一些极端情况,例如给定输入是否为负数或数字的反转超过 32 位。
为了进一步增强您对反转数字及其使用精美示意图的知识,您可能希望查看发表在C Program to Reverse a Number on Scaler 主题上的这篇精选文章。