在本教程中,我们将演示如何使用 react native 和 expo 播放视频。
在本文中,我们将实现如何使用 expo 创建 React Native 视频播放器。
如果您对 react native expo 视频播放器示例有疑问,那么我将给出简单的示例和解决方案。
您将学习如何使用 expo 在 react native 中暂停和播放视频。
您只需要一些步骤来完成如何在 react native expo 应用程序中添加播放视频。
让我们开始下面的例子:
第 1 步:下载项目
在第一步中运行以下命令来创建一个项目。
expo init ExampleApp
第 2 步:安装和设置
首先你必须安装 expo-av 包。
expo install expo-av
第 3 步:App.js
在这一步中,您将打开 App.js 文件并放置代码。
import * as React from 'react';
import { View, StyleSheet, StatusBar } from 'react-native';
import { Video } from 'expo-av';
const App = () => {
const video = React.useRef(null);
const [status, setStatus] = React.useState({});
return (
<View style={styles.container}>
<View style={styles.videoContainer}>
<Video
ref={video}
style={styles.video}
source={{
uri: 'https://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4',
}}
useNativeControls
resizeMode="cover"
isLooping
onPlaybackStatusUpdate={status => setStatus(() => status)}
/>
</View>
<StatusBar />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor: '#FFF',
padding: 10,
},
video: {
alignSelf: 'center',
width: '100%',
height: 400,
},
buttons: {
flexDirection: 'row',
justifyContent: 'center',
alignItems: 'center',
},
videoContainer: {
shadowColor: '#000',
shadowOffset: {
width: 0,
height: 1
},
shadowOpacity: 0.8,
shadowRadius: 2,
elevation: 2,
padding: 15,
},
});
export default App;
运行项目
在最后一步中,使用以下命令运行您的项目。
expo start
您可以在移动设备上的 Expo Go 应用程序中扫描二维码。
输出 :
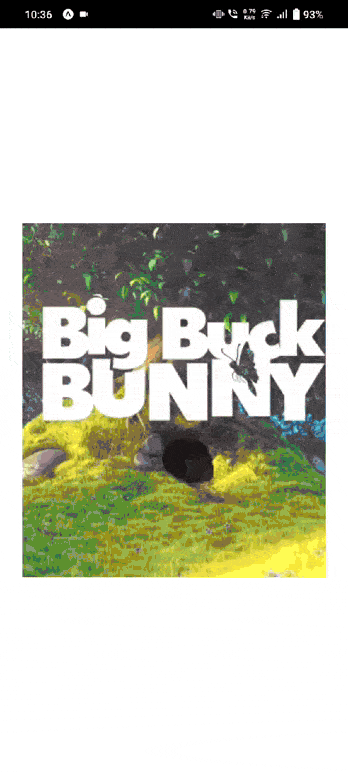
希望对您有用…
how-to-play-video-with-react-native-and-expo