如果您使用 .NET Core 编写代码,总有一天,您将不得不配置您的应用程序。
Microsoft在这里有很棒的文档。
我想与您分享编写普通应用程序真正需要的东西。我将使用 .NET Core 5。假设我们要创建一个简单的 Web API 项目。首先,您必须了解配置本身是由IConfiguration接口呈现的:
public interface IConfiguration
{
string this[string key] { get; set; }
IEnumerable<IConfigurationSection> GetChildren();
IChangeToken GetReloadToken();
IConfigurationSection GetSection(string key);
}
知道IConfiguration的实例已经注入Startup.cs文件是很有用的,您可以使用Configuration属性访问整个配置:
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
简单的例子
如果您需要连接字符串,这可能会正常工作。让我们使用appsetting.json文件,它是 .NET Core Web API 应用程序的默认设置。这是创建此类项目时生成的代码:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*"
}
假设我们要添加一个连接字符串部分。appsetting.json文件现在可能如下所示。这里的连接字符串只是一个示例。您可能会为您的数据库使用正确的数据库:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"DefaultConnection": "Server=YOURSERVERNAME; Database=YOURDATABASENAME; Trusted_Connection=True; MultipleActiveResultSets=true"
}
}
现在我们可以按如下方式访问连接字符串。假设我们有一些继承IUserService接口的UserService类,我们想用配置中的连接字符串实例化它:
public void ConfigureServices(IServiceCollection services)
{
var defaultConnectionString = Configuration.GetConnectionString("DefaultConnection");
services.AddScoped<IUserInterface>(x => new UserService(defaultConnectionString));
// another code follows here
}
高级示例
但是,使用整个配置实例可能会有点不知所措。更好的方法是引入自己的配置类。假设您需要将支付系统集成到您的应用程序中。以下是您需要执行的步骤。首先,我们需要在 appsettings.json 中添加一个新的PaymentOptions部分:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"DefaultConnection": "Server=YOURSERVERNAME; Database=YOURDATABASENAME; Trusted_Connection=True; MultipleActiveResultSets=true"
},
"PaymentOptions": {
"Host": "https://host.com",
"ApiKey": "hV79DIyA8WEH3AE2N1RDgVDHyEgTPnYC"
}
}
我们需要引入对应的C#类:
public class PaymentOptions
{
public string Host { get; set; }
public string ApiKey { get; set; }
}
现在我们可以在Startup.cs文件中注入PaymentOptions的一个实例:
public void ConfigureServices(IServiceCollection services)
{
// another code follows here
services.Configure<PaymentOptions>(Configuration.GetSection(nameof(PaymentOptions)));
// another code follows here
}
之后,我们可以在任何类构造函数中使用PaymentOptions的实例,通过默认的依赖注入机制访问支付选项。
如何为不同的环境设置不同的值?
默认情况下,会为新项目创建appsettings.json和appsettings.Development.json文件。这意味着您可以按照该模式为不同的环境创建配置文件并在它们之间切换。配置文件名的模式是appsettings.[Environment name].json。
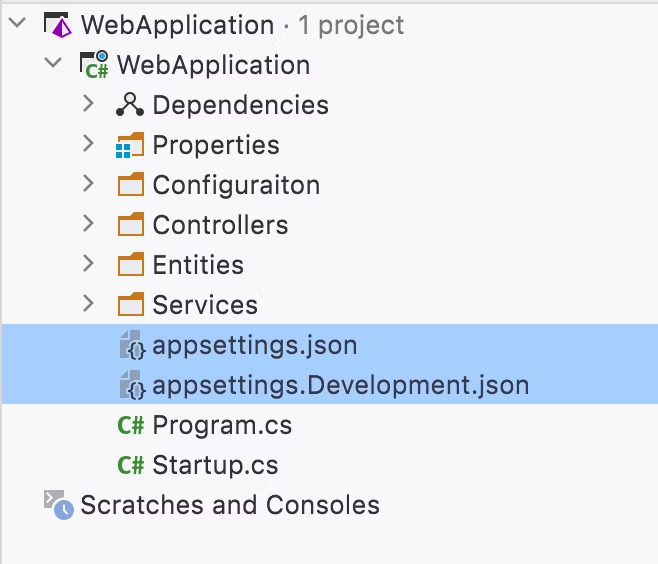
默认配置文件
您可以为不同文件中的配置属性设置不同的值。要在不同的环境配置之间切换,您必须设置ASPNETCORE_ENVIRONMENT环境变量。
如何添加自定义 JSON 文件?
有时,您需要添加具有自定义名称或路径的配置文件。它可以在Program.cs文件中轻松完成。您只需为 IHostBuilder 的实例调用ConifugureAppConfiguration ()方法。
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
// attach additional config JSON files
.ConfigureAppConfiguration((hostingContext, config) =>
{
config.AddJsonFile("secret/appsettings.secret.json", optional: true);
config.AddJsonFile("config/appsettings.json", optional: true, reloadOnChange: true);
})
.ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); });
有几件重要的事情需要注意,使用reloadOnChange属性意味着如果配置发生更改,平台将在运行时重新加载它。另一件事是配置文件可能是可选的。
概括
.NET Core 应用程序的配置非常简单,并且可以非常快速地完成。您可以使用 JSON 文件以结构良好的格式创建配置,并完全支持 .NET 平台,从而以自然的方式使用它。